我們使用 props 將信息/數(shù)據(jù)從父組件傳遞到子組件。在這篇文章中,我將解釋你需要知道的關(guān)于 props 的一切,以及為什么你應(yīng)該在 Vue.js 中使用 props。
以下是我們將在本指南中涵蓋的內(nèi)容的簡(jiǎn)要概述:
- Vue.js 中的 props 是什么?
- 如何在組件內(nèi)注冊(cè) props
- 如何使用多個(gè)props
- Vue.js 組件類型
- 如何將數(shù)據(jù)傳遞給 props
- 如何將函數(shù)傳遞給 props
- 如何驗(yàn)證props
- 如何為 props 設(shè)置默認(rèn)值
Vue.js 中的 Props 是什么?
“Props”是一個(gè)特殊的關(guān)鍵字,代表屬性。它可以在組件上注冊(cè)以將數(shù)據(jù)從父組件傳遞到其子組件之一。
與在 Vue.js 應(yīng)用程序中使用像 vuex 這樣的狀態(tài)管理庫(kù)相比,這要容易得多。
props 中的數(shù)據(jù)只能以一種方式流動(dòng)——從頂部或父組件,到底部或子組件。這只是意味著您不能將數(shù)據(jù)從子級(jí)傳遞給父級(jí)。
要記住的另一件事是 Props 是只讀的,不能被子組件修改,因?yàn)楦附M件“擁有”該值。
現(xiàn)在讓我們平衡一下——父組件將 props 傳遞給子組件,而子組件向父組件發(fā)出事件。
如何在組件內(nèi)注冊(cè)道具
現(xiàn)在讓我們看看如何在組件中注冊(cè) props。
Vue.component('user-detail', {
props: ['name'],
template: '<p>Hi {{ name }}</p>'
})
.js
或者,在 Vue 單文件組件中:
<template>
<p>{{ name }}</p>
</template>
<script>
export default {
props: ['name']
}
</script>
在上面的代碼中,我們注冊(cè)了一個(gè)名為的道具name,我們可以在我們的應(yīng)用程序的模板部分調(diào)用它。
注意:這是子組件,這個(gè)道具將從父組件接收數(shù)據(jù)。稍后我將詳細(xì)解釋這一點(diǎn)。
如何使用多個(gè)道具
你可以通過(guò)將它們附加到 props 數(shù)組來(lái)?yè)碛卸鄠€(gè) prop,就像這樣:
Vue.component('user-detail', {
props: ['firstName', 'lastName'],
template: '<p>Hi {{ firstName }} {{ lastName }}</p>'
})
或者,在 Vue 單文件組件中:
<template>
<p>Hi {{ firstName }} {{ lastName }}</p>
</template>
<script>
export default {
props: [
'firstName',
'lastName'
],
}
</script>
Vue.js 道具類型
要指定要在 Vue 中使用的 prop 類型,您將使用對(duì)象而不是數(shù)組。您將使用屬性名稱作為每個(gè)屬性的鍵,使用類型作為值。
如果傳遞的數(shù)據(jù)類型與 prop 類型不匹配,Vue 會(huì)在控制臺(tái)中發(fā)送警報(bào)(在開(kāi)發(fā)模式下)并帶有警告。您可以使用的有效類型是:
- 字符串
- 號(hào)碼
- 布爾值
- 數(shù)組
- 對(duì)象
- 日期
- 功能
- 符號(hào)
Vue.component('user-detail', {
props: {
firstName: String,
lastName: String
},
template: '<p>Hi {{ firstName }} {{ lastName }}</p>'
})
或者,在 Vue 單文件組件中:
<template>
<p>Hi {{ firstName }} {{ lastName }}</p>
</template>
<script>
export default {
props: {
firstName: String,
lastName: String
},
}
</script>
如何在 Vue 中將數(shù)據(jù)傳遞給 props
使用 props 的主要目標(biāo)是傳遞數(shù)據(jù)/信息。您可以使用 v-bind 將您的值作為數(shù)據(jù)屬性傳遞,例如在此代碼中:
<template>
<ComponentName :title=title />
</template>
<script>
export default {
//...
data() {
return {
title: 'Understanding Props in vuejs'
}
},
//...
}
</script>
或作為這樣的靜態(tài)值:
<ComponentName title="Understanding Props in vuejs" />
假設(shè)我們正在構(gòu)建一個(gè)應(yīng)用程序,其中包含許多具有不同文本/背景顏色的按鈕。與其在我們的所有文件中重復(fù)按鈕語(yǔ)法,不如創(chuàng)建一個(gè)按鈕組件,然后將文本/背景顏色作為道具傳遞。
這是父組件:
<template>
<div id="app">
<Button :name='btnName' bgColor='red' />
<Button :name='btnName' bgColor='green' />
<Button :name='btnName' bgColor='blue' />
</div>
</template>
<script>
import Button from './components/Button'
export default {
name: 'App',
data(){
return{
btnName:"Joel",
}
},
components: {
Button
}
}
</script>
這是子組件:
<template>
<button class="btn" :style="{backgroundColor:bgColor}">{{name}}</button>
</template>
<script>
export default {
name: 'Button',
props:{
name:String,
bgColor:String
}
}
</script>
上面的代碼向您展示了當(dāng)您從父組件獲取數(shù)據(jù)并在子組件中使用該數(shù)據(jù)時(shí)如何使用數(shù)據(jù)屬性和靜態(tài)值。
注意:您還可以在 prop 值中使用三元運(yùn)算符來(lái)檢查真實(shí)條件并傳遞依賴于它的值。
<template>
<div id="app">
<Button :tagUser="signedUp ? 'Logout' : 'Login'" bgColor='red' />
</div>
</template>
<script>
import Button from './components/Button'
export default {
name: 'App',
data(){
return{
signedUp: true,
}
},
components: {
Button
}
}
</script>
在上面的代碼中,我們正在檢查signedUp數(shù)據(jù)屬性。如果是true,發(fā)送的數(shù)據(jù)應(yīng)該是Logout, 否則應(yīng)該是Login。
如何將函數(shù)傳遞給 props
將函數(shù)或方法作為 prop 傳遞給子組件相對(duì)簡(jiǎn)單。它與傳遞任何其他變量的過(guò)程基本相同。
但是你不應(yīng)該使用 props 作為函數(shù)是有原因的——你應(yīng)該使用emit。
<template>
<ChildComponent :function="newFunction" />
</template>
<script>
export default {
methods: {
newFunction() {
// ...
}
}
};
</script>
如何在 Vue 中驗(yàn)證 props
Vue 讓驗(yàn)證 props 變得非常容易。您所要做的就是將所需的鍵及其值添加到道具中。我們可以使用 prop 類型和使用來(lái)驗(yàn)證required:
props: {
name: {
type: String,
required: true
}
}
如何設(shè)置默認(rèn)道具值
在結(jié)束本文之前,讓我們現(xiàn)在看看如何為我們的道具設(shè)置默認(rèn)值。如果子組件無(wú)法從父組件獲取數(shù)據(jù),則呈現(xiàn)默認(rèn)值。
Vue 允許你指定一個(gè)默認(rèn)值,就像我們r(jià)equired之前指定的那樣。
props: {
name: {
type: String,
required: true,
default: 'John Doe'
},
img: {
type: String,
default: '../image-path/image-name.jpg',
},
}
您還可以將默認(rèn)值定義為對(duì)象。它可以是一個(gè)返回適當(dāng)值的函數(shù),而不是實(shí)際值。
結(jié)論
在本文中,我們學(xué)習(xí)了 props 的作用以及 props 在 Vue.js 中是如何工作的。
總之,我們使用 props 將數(shù)據(jù)從父組件傳遞給子組件。子組件還會(huì)向父組件發(fā)出事件,以防您需要從子組件向父組件發(fā)送數(shù)據(jù)/事件。
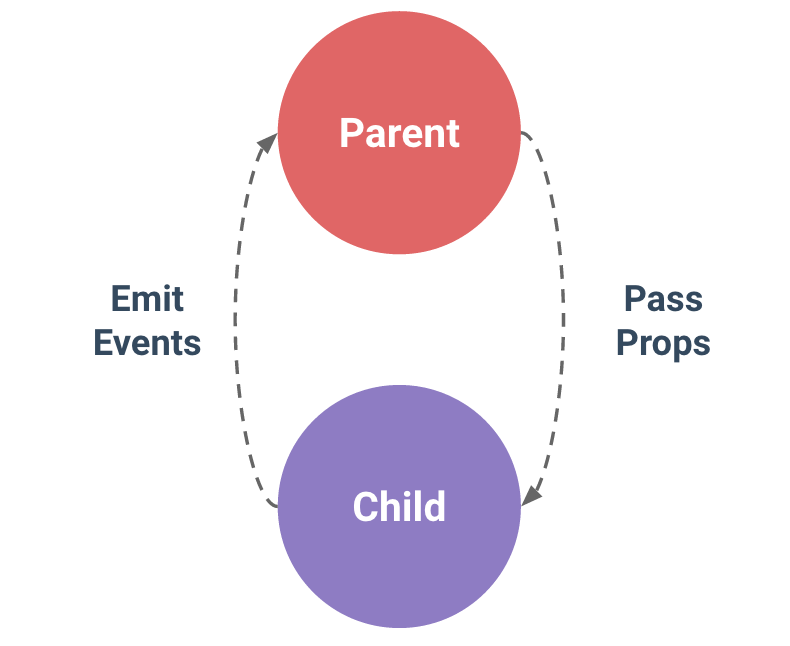
感謝您的閱讀!